Advanced Team View Filters
Team View Filters allow supervisors to search or filter their agents by name or activity. You can also use custom, programmatically defined filter criteria, like teams or skills. This page describes the main concepts involved in customizing Team View Filters, and includes some sample code you can use to get started with the feature. For more information about using Team View Filters, check out the End User Guide!
Warning
This feature is in pilot between version 1.18 and version 1.26.3 of the Flex UI. You can enable team view filters on the pre-release features page within Flex Admin. This feature is enabled by default for version 1.27.0 and upper.
Flex uses the following objects and components to generate Team View Filters:
The portion of the Flex UI that shows the Team Filters. It is a child component of the Supervisor UI. By default, Flex allows you to filter by agent Activity, but you can add custom filters to the panel by modifying the defaultProps, like so:
1Flex.TeamsView.defaultProps.filters = [2Flex.TeamsView.activitiesFilter,3// ... custom filters here4];5
A FilterDefinition is a structure that tells Flex how to render the new filter label and field, and what to query the field value against. Each field will add a new condition to the Sync query that will render the agents (or workers) list to the UI. A FilterDefinition has the following structure:
1interface FilterDefinition {2id: string;3title: React.ReactChild;4fieldName: string;5type?: FiltersListItemType;6options?: Array;7customStructure?: CustomFilterItemStructure;8condition?: string;9}10
Field | Description |
---|---|
id | Required. A string representing the subject of your query. This identifies the values to filter for in the Flex app state based on your specified attribute or field. For example, "data.attributes.full_name" or "data.activity_name". |
title | Required. A string that is rendered as the filter display name on the Filters side panel. For example, "Names". |
fieldName | Required. A string representing the input name passed to the predefined filters. For example, "full_name". |
type | Required if customStructure isn't defined. Currently only supports "multiValue". This renders a list of checkboxes for each option you provide. |
options | Required if type is "multiValue". An array of filter definition options used for rendering the checkbox fields, their values, their labels, and whether they are selected by default. |
customStructure | Required if type isn't set. An object of type CustomFilterItemStructure used for rendering custom fields. This lets you add your own custom fields. |
condition | An optional parameter that represents the query comparison operator such as IN , NOT_IN , CONTAINS . See Query Operators to learn about other possible condition values. This represents the condition to be used in building the query. For instance, data.activity_name IN ["available"] or data.attributes.full_name CONTAINS "John" . In the latter example, "data.attributes.full_name" is the id , "CONTAINS" is the condition , and "John" is the value to filter for. |
Describes the available options which can be used as a filter. For example, if you wanted to filter by agent languages, you could create an option for each language spoken in your contact center.
1interface FilterDefinitionOption {2value: string;3label: string;4default?: boolean;5}
Field | Description |
---|---|
value | Value of the filter option. When using IN or NOT_IN to build your query and you are providing an array of options, you need to omit the first and the last instances of double quotes in your string. See example in Add a multivalue field |
label | Friendly name of the option. |
default | A Boolean value indicating whether the filter option should be selected by default. |
To add a custom field and label to a filter, you'll need an object with a select field or an input field passed to the CustomFilterItemStructure
.
1interface CustomFilterItemStructure {2field: React.ReactElement;3label: React.ReactElement;4}5
field
is a React element that should render an input usable by the final customer. It inherits a few custom props from Flex.
Field | Description |
---|---|
name | The field name set in the FilterDefinition. |
handleChange | A function that gets invoked on this custom field change event. It requires the new value of the filter to be passed as an argument, either as an array of strings or a string. |
options | The same options passed in the FilterDefinition, if provided. |
currentValue | The current value of the filter. It can either be an array of strings or a string, depending on what the props.handleChange function receives once invoked. |
label is another React element that serves as the label of the filter. It should indicate the content of the filter when the accordion item is closed. It receives the following properties:
Field | Description |
---|---|
currentValue | The current value of the filter. |
activeOption | is the options array that is provided and returns the entire selected option. It will contain currentValue as value. |
The filters array also accepts FiltersDefinitionFactories, which are functions that return a FilterDefinition. You can write a FilterDefinitionFactory that fetches data from the app state and dynamically renders values or options of a field.
Your factory must accept two arguments:
Argument | Description |
---|---|
appState | The entire Flex Redux state. Use this to access information about activities, session, and more details about the current state of your Flex instance. |
ownProps | The props received by the TeamFiltersPanel. |
The TeamFiltersPanel includes activitiesFilter
by default. To add a new filter to the TeamsFiltersPanel, you can overwrite the defaultProps.filters
property with a new array of filter definitions before rendering your Flex instance.
This array can contain both FilterDefinitions and FilterDefinition factories. In this example, we are reading the default activity filter as the first item of the array.
1Flex.TeamsView.defaultProps.filters = [2Flex.TeamsView.activitiesFilter,3yourFilterDefinitionHere,4your2ndFilterDefinitionHere,5your3rdFilterDefinitionHere,6];7
Initially, the FilterDefinition has one predefined type, which will output checkboxes: MultiValue. With custom input fields, you can add a different type of input, like a custom text input field.
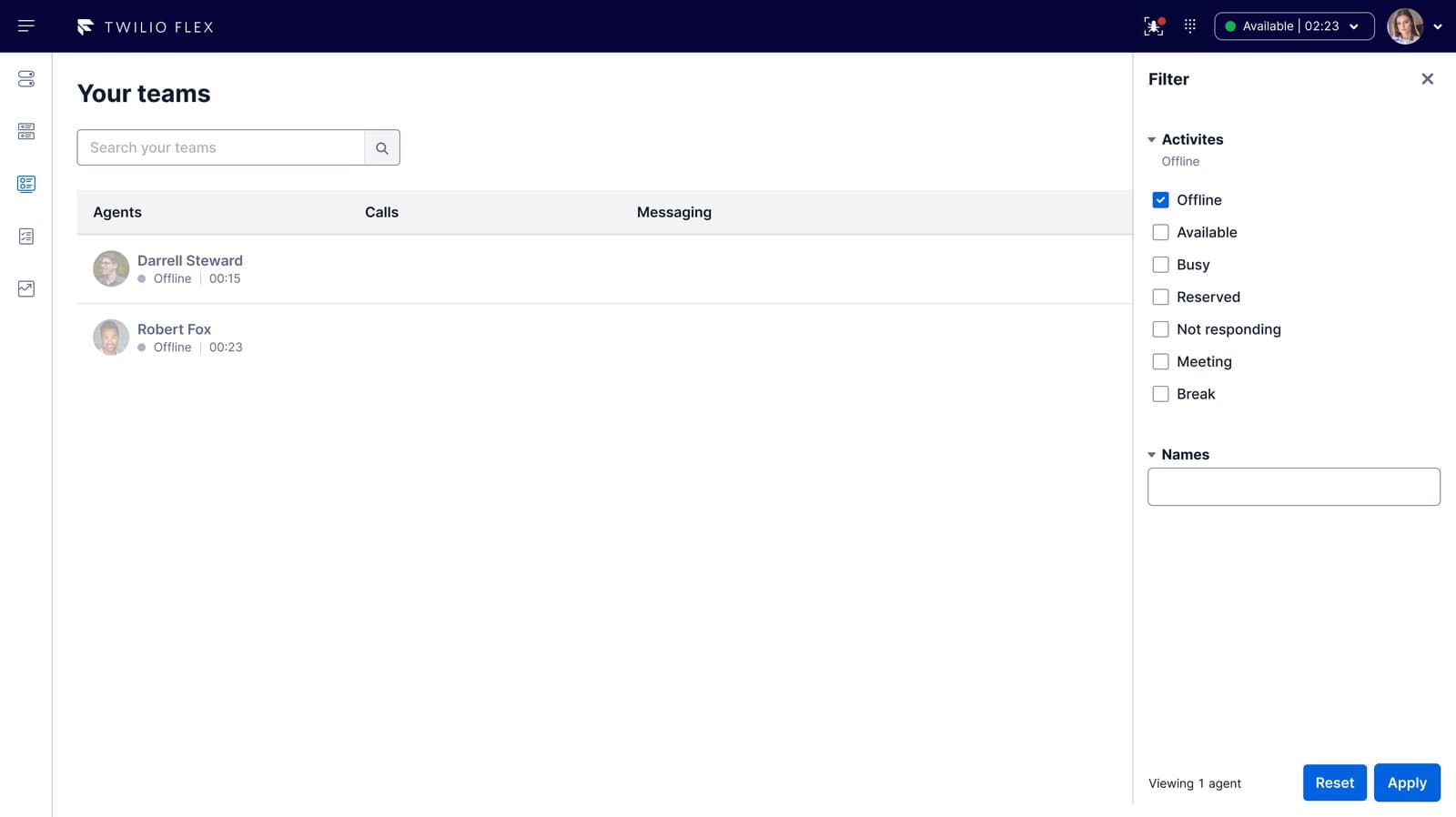
An example of a custom input field, name, where you can type and filter by names based on the condition that you specify
The following file describes the HTML for your custom field, as well as the JavaScript associated with its behavior. In this sample, the filter functionality is extended in the extendFilters function
1import React from "react";2import { TeamsView } from "@twilio/flex-ui";34// Define an Input component; returns an input HTML element with logic to handle changes when users enter input5const Input = ({ handleChange, currentValue = "", fieldName }) => {6const _handleChange = (e) => {7e.preventDefault();8handleChange(e.target.value);9};return (10<input11className="CustomInput"12type="text"13onChange={_handleChange}14value={currentValue}15name={fieldName}16/>17)18};1920// Define the label that supervisors will see when using our custom filter21const CustomLabel = ({ currentValue }) => (22<>{currentValue && currentValue.length ? `Containing "${currentValue}"` : "Any"}</>23);2425// Define a new filter that uses the custom field26const nameFilter = {27id: "data.attributes.full_name",28fieldName: "full_name",29title: "Names",30customStructure: {31field: <Input />,32label: <CustomLabel />,33},34condition: "CONTAINS"35};3637// Export a function to be invoked in plugin's main entry-point38export const extendFilter = (manager) => {39manager.updateConfig({40componentProps: {41TeamsView: {42filters:[43TeamsView.activitiesFilter,44nameFilter,45]46}47}48})49};
The following example will filter by agents located in any of the options specified in the value
field:
1import React from "react";2import { TeamsView } from "@twilio/flex-ui";3import { FiltersListItemType } from "@twilio/flex-ui";45// Define a new filter that uses the custom field67const teamFilter = {8fieldName: "team_location",9title: 'Team',10id: 'data.attributes.squad',11type: FiltersListItemType.multiValue,12options: [13{ label: 'Canada', value: 'CAN-1", "CAN-2", "ARC'}14],15condition: 'IN',16};1718// Export a function to be invoked in plugin's main entry-point19export const extendFilter = (manager) => {20manager.updateConfig({21componentProps: {22TeamsView: {23filters: [24TeamsView.activitiesFilter,25teamFilter,26]27}28}29})30};
You can use a filter definition factory to dynamically render the options of the predefined checkboxes fields. For example, you could render the activities that are currently available on in the Flex Redux store.
1import React from "react";23import { FiltersListItemType } from "@twilio/flex-ui";45const customActivityFilter = (appState, teamFiltersPanelProps) => {6const activitiesArray = Array.from(appState.worker.activities.values());78const activities = (activitiesArray).map((activity) => ({9value: activity.name,10label: activity.name,11default: !!activity.available,12}));1314return {15id: "data.activity_name",16fieldName: "custom_activity_name",17type: FiltersListItemType.multiValue,18title: "Activities",19options: activities20};21};2223// Export a function to be invoked in plugin's main entry-point24export const extendFilter = (manager) => {25manager.updateConfig({26componentProps: {27TeamsView: {28filters: [29customActivityFilter,30]31}32}33})34};
You can use custom filter input components and filter definition factories together. The following code sample dynamically renders the options of a select field from the current Redux store.
1import React from "react";23// Create your custom field4const CustomField = ({ handleChange, currentValue, fieldName, options }) => {5const _handleChange = (e) => {6e.preventDefault();7handleChange(e.target.value);8};910return (11<select12className="CustomInput"13onChange={_handleChange}14value={currentValue}15name={fieldName}16>17<option value="" key="default">All activities</option>18{options.map(opt => (19<option value={opt.value} key={opt.value}>{opt.label}</option>20))}21</select>22)23};2425// Define the label that will be displayed when filter is active26const CustomLabel = ({ currentValue }) => (27<>{currentValue || "All activities"}</>28);2930// Define the available properties upon which to filter based on application state31const customActivityFilter = (appState, teamFiltersPanelProps) => {3233const activitiesArray = Array.from(appState.worker.activities.values());3435const activities = (activitiesArray).map((activity) => ({36value: activity.name,37label: activity.name,38default: !!activity.available,39}));4041return {42id: "data.activity_name",43fieldName: "custom_activity_name",44title: "Activities",45customStructure: {46label: <CustomLabel />,47field: <CustomField />,48},49options: activities50};51};5253// Add new filter to TeamFiltersPanel in the Flex UI54export const extendFilter = (manager) => {55manager.updateConfig({56componentProps: {57TeamsView: {58filters: [59customActivityFilter,60]61}62}63})64};
Once you've configured your filter(s), you can enable them in Flex manager from within the base Plugin file. In this example, the extendFilters
function is imported and passed to the Flex Manager.
1import Flex from '@twilio/flex-ui';2import { FlexPlugin } from 'flex-plugin';3import { extendFilter } from "./CustomFilters";45const PLUGIN_NAME = 'SamplePlugin';67export default class SamplePlugin extends FlexPlugin {8constructor() {9super(PLUGIN_NAME);10}1112/**13*14* @param flex15* @param {Manager} manager16*/17init(flex, manager) {18extendFilter(manager);19}20}
The following code samples demonstrate writing and calling the same extendFilters
functions, but in the context of self-hosted Flex.
1import React from "react";2import Flex from "@twilio/flex-ui";34// Define an Input component; returns a simple input HTML element with logic to handle changes when users enter input5const Input = ({ handleChange, currentValue = "", fieldName }) => {6const _handleChange = (e) => {7e.preventDefault();8handleChange(e.target.value);9};1011return (12<input13className="CustomInput"14type="text"15onChange={_handleChange}16value={currentValue}17name={fieldName}18/>19)20};2122// Define the label that supervisors will see when using our custom filter23const CustomLabel = ({ currentValue }) => (24<>{currentValue && currentValue.length ? `Containing "${currentValue}"` : "Any"}</>25);262728// Define a new filter that uses the custom field29const nameFilter = {30id: "data.attributes.full_name",31fieldName: "full_name",32title: "Names",33customStructure: {34field: <Input />,35label: <CustomLabel />,36},37condition: "CONTAINS"38};3940// Add the filter to the list of filters in the TeamFiltersPanel41export const extendFilter = () => {42Flex.TeamsView.defaultProps.filters = [43Flex.TeamsView.activitiesFilter,44nameFilter,45];46};
1import React from "react";2import Flex from "@twilio/flex-ui";34const customActivityFilter = (appState, teamFiltersPanelProps) => {5const activitiesArray = Array.from(appState.worker.activities.values());67const activities = (activitiesArray).map((activity) => ({8value: activity.name,9label: activity.name,10default: !!activity.available,11}));1213return {14id: "data.activity_name",15fieldName: "custom_activity_name",16type: Flex.FiltersListItemType.multiValue,17title: "Activities",18options: activities19};20};212223export const extendFilter = () => {24Flex.TeamsView.defaultProps.filters = [25customActivityFilter,26];27};
1import React from "react";2import Flex from "@twilio/flex-ui";34// Create your custom field5const CustomField = ({ handleChange, currentValue, fieldName, options }) => {6const _handleChange = (e) => {7e.preventDefault();8handleChange(e.target.value);9};1011return (12<select13className="CustomInput"14onChange={_handleChange}15value={currentValue}16name={fieldName}17>18<option value="" key="default">All activities</option>19{options.map(opt => (20<option value={opt.value} key={opt.value}>{opt.label}</option>21))}22</select>23)24};2526// Define the label that will be displayed when filter is active27const CustomLabel = ({ currentValue }) => (28<>{currentValue || "All activities"}</>29);3031// Define the available properties upon which to filter based on application state32const customActivityFilter = (appState, teamFiltersPanelProps) => {3334const activitiesArray = Array.from(appState.worker.activities.values());3536const activities = (activitiesArray).map((activity) => ({37value: activity.name,38label: activity.name,39default: !!activity.available,40}));4142return {43id: "data.activity_name",44fieldName: "custom_activity_name",45title: "Activities",46customStructure: {47label: <CustomLabel />,48field: <CustomField />,49},50options: activities51};52};5354// Add new filter to TeamFiltersPanel in the Flex UI55export const extendFilter = () => {56Flex.TeamsView.defaultProps.filters = [57customActivityFilter,58];59};
1import { extendFilter } from "./CustomFilters";23export function run(config) {4const container = document.getElementById("container");56return Flex7.progress("#container")8.provideLoginInfo(config, "#container")9.then(() => Flex.Manager.create(config))10.then(manager => {1112// Extending the filter functionality13extendFilter();1415ReactDOM.render(16<Flex.ContextProvider manager={manager}>17<Flex.RootContainer />18</Flex.ContextProvider>,19container20);21})22.catch((e) => {23console.log("Failed to run Flex", e);24});25}